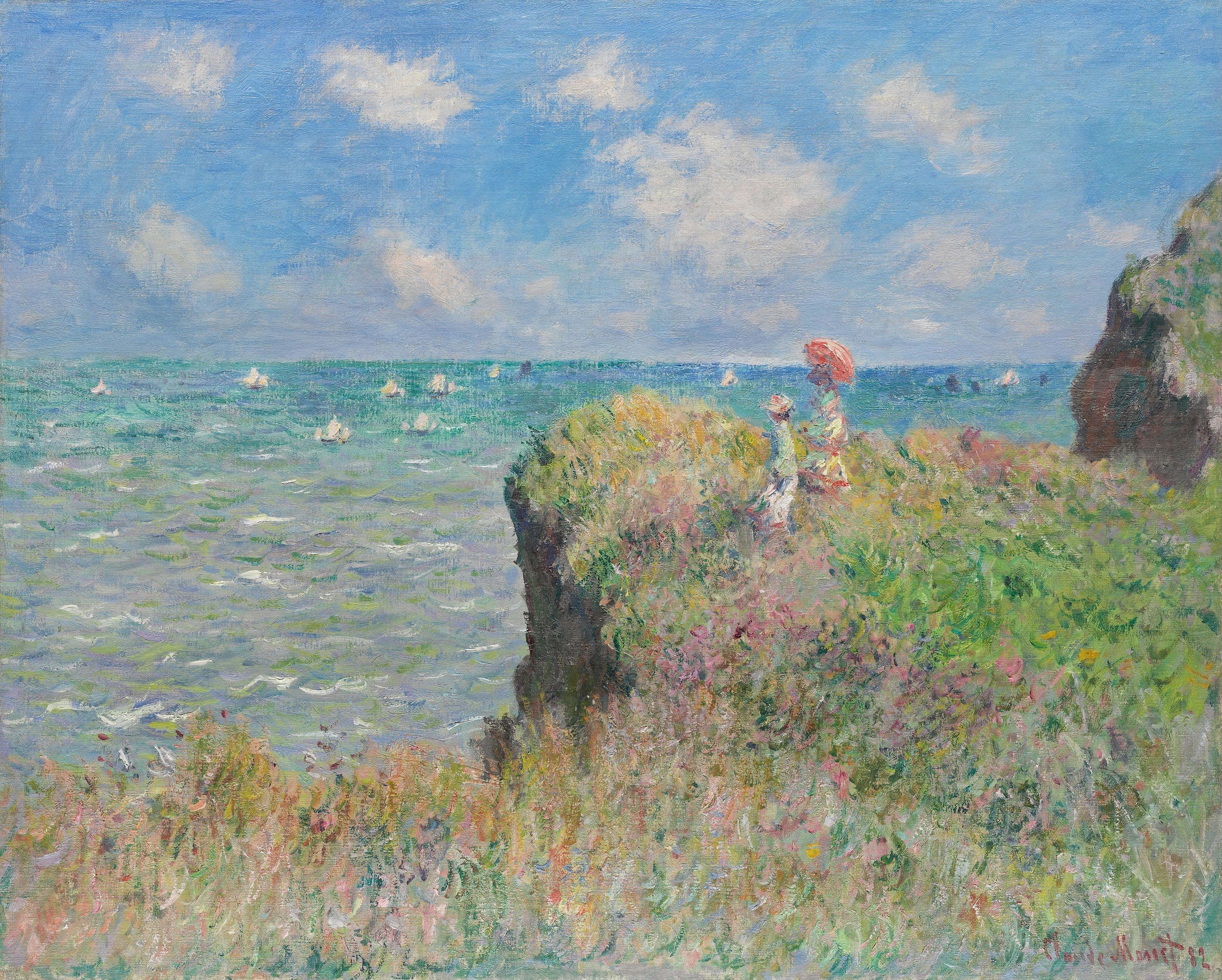
Building a blog with Astro and Sveltia CMS
January 30, 2025
I've been a SvelteKit fan for a couple of years, but I encountered a few issues with client side routing. They might be fixed by now, but I just wanted to switch to something simpler. No client side routing is needed for me, hey, I'm just making my blog. For the content management system, well, I used Decap CMS for udvarfeszt.hu, which fitted very well into my GitHub CI/CD based workflow, but we encountered some issues: besides some performance problems, their Slate editor (for markdown editing) just crashes sometimes (seems like the bug is still there), which is a critical-critical bug, so I decided to try switching to Sveltia CMS instead, which prides itself with being mostly compatible with Decap CMS. Yay. So I decided to just build my blog using Astro and Sveltia CMS, and if all works, I can try migrating udvarfeszt.hu too.
And the plan worked out. Honestly, using Astro with Sveltia CMS was an amazing experience. The two components worked well out of the box, so most of the work was gluing them together and coding the website. Let's focus on the gluing part. I exported everything as JSON from Sveltia CMS*, and JSON can be imported directly when using Vite. After importing, we can display the values of the different fields in the JSON objects., but first, the field value has to be treated based on its field type, with the most interesting and challenging one being the Markdown field.
One of the most straightforward ways of displaying Markdown is converting Markdown to HTML and then injecting it using innerHTML (known as set:html in Astro). Normal Markdown to HTML is easy, I just use marked or something. However, I wanted to use Astro's Image/Picture component so that I can use their nice automatic progressive enhancement. And luckily, their experimental_AstroContainer supports rendering Astro components as HTML. Woo-hoo! Here's the code for transpiling Markdown to HTML:
import { experimental_AstroContainer } from 'astro/container'
import { marked, type MarkedExtension } from 'marked'
import Pic from 'src/layouts/Pic.astro'
import hljs from 'highlight.js'
const container = await experimental_AstroContainer.create()
const uploads = import.meta.glob('/src/assets/uploads/**', {
eager: true
})
const myExtension: MarkedExtension = {
async: true,
async walkTokens(token) {
if (token.type === 'image') {
token.type = 'html'
token.text = await container.renderToString(Pic, {
props: {
src: (uploads[token.href] as any).default,
alt: token.text || '',
title: token.title || '',
sizes: '(max-width: 400px) 370px, 740px',
loading: 'lazy'
} as any
})
}
if (token.type === 'code') {
const lang = token.lang || 'plaintext'
const code = token.text
const language = hljs.getLanguage(lang) ? lang : 'plaintext'
token.type = 'html'
token.text = `<pre><code class="hljs hljs-${language}">${hljs.highlight(code, { language }).value}</code></pre>`
}
}
}
marked.use(myExtension)
export async function parseMarkdown(content: string): Promise<string> {
return marked.parse(content)
}
*I think exporting posts as Markdown would have been easier in the case of my website, because Astro supports directly using Markdown files as pages, but there would be a problem with that in the case of udvarfeszt.hu: These Markdown pages only support one Markdown field, so on urdvarfeszt.hu, I would need to find a way to render Markdown manually anyway. I figured, then I might as well just export everything as JSON to reduce complexity - then I just handle these JSON files, and that's it. Vite supports importing then, and I even get nice autocompletion, so I only have to figure out a way to render Markdown as HTML.